C# Program how to handle exceptions:
Object: Write a C sharp program to illustrate try-catch action with the following Exceptions. C# Projects with Source Code
i. IndexOutOfRangeException
ii. ArgumentOutOfRangeException
iii. ArrayTypeMismatchException
Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace exc
{
class exp1
{
public void pro1()
{
string[] str = new string[4];
str[0] = “Let us C”;
str[1] = “Basic C++”;
str[2] = “C# book for beginners”;
str[3] = “Python”;
try
{
Console.WriteLine(“\n—IndexOutOfRangeException—\n”);
Console.WriteLine(“Enter Book Name. “);
string nam = Console.ReadLine();
for (int i = 0; i < 6; i++)
{
if (nam == str[i])
{
Console.WriteLine(“\nBook is Available in Library”);
break;
}
}
}
catch (IndexOutOfRangeException e)
{
Console.WriteLine(“\nThe book is Already issued!!! ” + e.Message);
}
finally
{
Console.WriteLine(“\n—ArgumentOutOfRangeException—\n”);
}
}
}
class exp2
{
public void pro2()
{
int num;
Console.WriteLine(“Enter Book Number.”);
num = Convert.ToInt32(Console.ReadLine());
try
{
if (num > 10)
{
throw new ArgumentOutOfRangeException();
}
else
{
Console.WriteLine(“Book Number is :” + num);
}
}
catch (ArgumentOutOfRangeException e2)
{
Console.WriteLine(“Error!! ” + e2.Message);
}
finally
{
Console.WriteLine(“\n—ArrayTypeMismatchException—\n”);
}
}
}
class exp3
{
public void pro3()
{
string[] str = { “Book 1”, “Book 2″,”Book 3” };
object[] ob = (object[])str;
try
{
object obj = (object)15;
ob[2] = obj;
}
catch (ArrayTypeMismatchException e3)
{
Console.WriteLine(“\nError!!! ” + e3.Message);
}
finally
{
Console.WriteLine(“\nLearn Programming with codeboks.com”);
}
}
}
class Program
{
static void Main(string[] args)
{
exp1 e1 = new exp1();
e1.pro1();
exp2 e2 = new exp2();
e2.pro2();
exp3 e3 = new exp3();
e3.pro3();
Console.ReadKey();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace exc
{
class exp1
{
public void pro1()
{
string[] str = new string[4];
str[0] = "Let us C";
str[1] = "Basic C++";
str[2] = "C# book for beginners";
str[3] = "Python";
try
{
Console.WriteLine("\n---IndexOutOfRangeException---\n");
Console.WriteLine("Enter Book Name. ");
string nam = Console.ReadLine();
for (int i = 0; i < 6; i++)
{
if (nam == str[i])
{
Console.WriteLine("\nBook is Available in Library");
break;
}
}
}
catch (IndexOutOfRangeException e)
{
Console.WriteLine("\nThe book is Already issued!!! " + e.Message);
}
finally
{
Console.WriteLine("\n---ArgumentOutOfRangeException---\n");
}
}
}
class exp2
{
public void pro2()
{
int num;
Console.WriteLine("Enter Book Number.");
num = Convert.ToInt32(Console.ReadLine());
try
{
if (num > 10)
{
throw new ArgumentOutOfRangeException();
}
else
{
Console.WriteLine("Book Number is :" + num);
}
}
catch (ArgumentOutOfRangeException e2)
{
Console.WriteLine("Error!! " + e2.Message);
}
finally
{
Console.WriteLine("\n---ArrayTypeMismatchException---\n");
}
}
}
class exp3
{
public void pro3()
{
string [] str = { "Book 1", "Book 2","Book 3" };
object [] ob = (object [])str;
try
{
object obj = (object)15;
ob[2] = obj;
}
catch (ArrayTypeMismatchException e3)
{
Console.WriteLine("\nError!!! " + e3.Message);
}
finally
{
Console.WriteLine("\nLearn Programming with codeboks.com");
}
}
}
class Program
{
static void Main(string[] args)
{
exp1 e1 = new exp1();
e1.pro1();
exp2 e2 = new exp2();
e2.pro2();
exp3 e3 = new exp3();
e3.pro3();
Console.ReadKey();
}
}
}
Output:
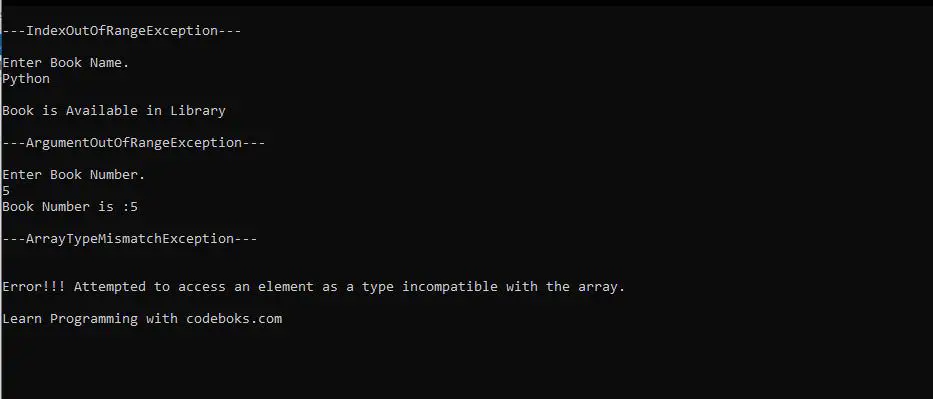

Pingback: Top 20 C# programs examples in 2021