Object: Write a C# program of throwing an exception when dividing by zero condition occurs. C# Projects with Source Code.
Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace code
{
class Program
{
static void Main(string[] args)
{
double num1, num2, divide;
try
{
Console.WriteLine(“Enter Number a : “);
num1 = Convert.ToDouble(Console.ReadLine());
Console.WriteLine(“Enter Number b : “);
num2 = Convert.ToDouble(Console.ReadLine());
divide = num1 / num2;
Console.WriteLine(“Value of a/b is : ” + divide);
}
catch (DivideByZeroException e)
{
Console.WriteLine(“Error : ” + e.Message);
}
finally
{
Console.WriteLine(“\nCodeboks.com”);
}
Console.ReadKey();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace code
{
class Program
{
static void Main(string[] args)
{
double num1, num2, divide;
try
{
Console.WriteLine("Enter Number a : ");
num1 = Convert.ToDouble(Console.ReadLine());
Console.WriteLine("Enter Number b : ");
num2 = Convert.ToDouble(Console.ReadLine());
divide = num1 / num2;
Console.WriteLine("Value of a/b is : " + divide);
}
catch (DivideByZeroException e)
{
Console.WriteLine("Error : " + e.Message);
}
finally
{
Console.WriteLine("\nCodeboks.com");
}
Console.ReadKey();
}
}
}
Output:

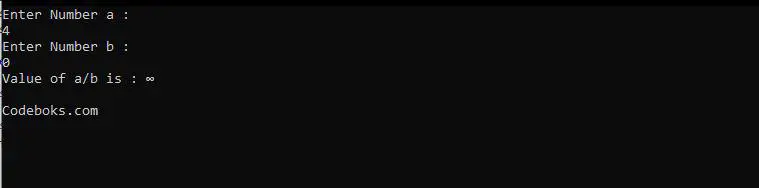
Another C# Program: C# Program Calculating the Area of a Rectangle
Another C# Program: C# Program that Subtracts two user-defined numbers
Another C# Program: C# program to count vowels in a string
Another Program: Write a C# program that reads two arrays and checks whether they are equal.
Another Program: Develop a C# app that will determine the gross pay for each of three employees