Write a Program Snake game in C++
How to make a game in C++:
This game will be made using ‘C++’ programming language. We’ll be creating this game using the ‘Console Mode’ window. Here, we’re looking at how to play the game and also making the game more interesting by adding some more advanced codes.
What do you mean by the Snake game?
The C++ snake game is a great game that tests the coordination between your eyes, hands and the brain in such a unique and fun way. The game is inspired by the old classic video game called “snake”. To play the game, you need to control your snake with your hands and tilt your phone to avoid the obstacles. The goal is to gather as many eggs as possible and also destroy the eggs of your opponents.
Object: Make a snake game in c++:
Write a Snake game Program in C++, C++ Projects.
Code:
#include<iostream>
#include<conio.h>
#include<windows.h>
using namespace std;
enum eDirection { STOP=0,LEFT,RIGHT,UP,DOWN};
eDirection dira;
bool gameOver;
void setup();
void Draw();
void Input();
void Logic();
void Logic2();
const int width=20;
const int height=20;
int x,y, fruitX, fruitY,score;
int tailx[100],taily[100];
int ntail;
int main()
{
char ch;
cout<<“\n\t—————————————\n”;
cout<<“\t\t:Snake game in C++:\n”;
cout<<“\t—————————————\n\n”;
cout<<“Please Enter Game Mod which you Play:\n\n”;
cout<<“Play Hard Mode for Press : H\n\n”;
cout<<“Play Easy for Press: S\n\n”;
cin>>ch;
switch (ch)
{
case ‘S’:
setup();
while(!gameOver)
{
Draw();
Input();
Logic();
_sleep(30);
}
break;
case ‘H’:
setup();
while(!gameOver)
{
Draw();
Input();
Logic2();
_sleep(30);
}
break;
default:
cout<<“Invalid!! Please Select Given Options.”;
break;
}
getch();
return 0;
}
void setup()
{
gameOver=false;
dira=STOP;
x=width/2;
y=height/2;
fruitX=rand()%width;
fruitY=rand()%height;
score=0;
}
void Draw()
{
system(“cls”);
cout<<“\n\t\t :SNAKE GAME:\n”;
cout<<“\t\t”;
for(int i=0;i<width-8;i++)
{
cout<<“||”;
}
cout<<endl;
for(int i=0;i<height;i++)
{
for(int j=0;j<width;j++)
{
if(j==0)
{
cout<<“\t\t||”;
}
if(i==y&&j==x)
cout<<“O”;
else if(i==fruitY&&j==fruitX)
cout<<“*”;
else
{
bool print=false;
for(int k=0;k<ntail;k++)
{
if(tailx[k]==j&& taily[k]==i)
{
cout<<“o”;
print=true;
}
}
if(!print)
{
cout<<” “;
}
}
if (j==width-1)
cout<<“||”;
}
cout<<endl;
}
cout<<“\t\t”;
for(int i=0;i<width-8;i++)
{
cout<<“||”;
}
cout<<endl;
cout<<“\t\t\tScore: “<<score<<endl;
}
void Input()
{
if(_kbhit())
{
switch(_getch())
{
case ‘a’:
dira=LEFT;
break;
case ‘d’:
dira=RIGHT;
break;
case ‘w’:
dira=UP;
break;
case ‘s’:
dira=DOWN;
break;
case ‘x’:
gameOver=true;
break;
}
}
}
//simple mode
void Logic()
{
int prevX=tailx[0];
int prevY=taily[0];
int prev2X,prev2Y;
tailx[0]=x;
taily[0]=y;
for(int i=1;i<ntail;i++)
{
prev2X=tailx[i];
prev2Y=taily[i];
tailx[i]=prevX;
taily[i]=prevY;
prevX=prev2X;
prevY=prev2Y;
}
switch (dira)
{
case LEFT:
x–;
break;
case RIGHT:
x++;
break;
case UP:
y–;
break;
case DOWN:
y++;
break;
default:
break;
}
if(x>=width) x=0; else if(x<0)x=width-1;
if(y>=height) y=0; else if(y<0)y=height-1;
for(int i=0;i<ntail;i++)
{
if(tailx[i]==x && taily[i]==y)
{
gameOver=true;
}
}
if(x==fruitX&&y==fruitY)
{
score+=10;
fruitX=rand()%width;
fruitY=rand()%height;
ntail++;
}
}
//hard mod
void Logic2()
{
int prevX=tailx[0];
int prevY=taily[0];
int prev2X,prev2Y;
tailx[0]=x;
taily[0]=y;
for(int i=1;i<ntail;i++)
{
prev2X=tailx[i];
prev2Y=taily[i];
tailx[i]=prevX;
taily[i]=prevY;
prevX=prev2X;
prevY=prev2Y;
}
switch (dira)
{
case LEFT:
x–;
break;
case RIGHT:
x++;
break;
case UP:
y–;
break;
case DOWN:
y++;
break;
default:
break;
}
if(x>width||x<0||y>height||y<0)
{
gameOver=true;
}
if(x>=width) x=0; else if(x<0)x=width-1;
if(y>=height) y=0; else if(y<0)y=height-1;
for(int i=0;i<ntail;i++)
{
if(tailx[i]==x && taily[i]==y)
{
gameOver=true;
}
}
if(x==fruitX&&y==fruitY)
{
score+=10;
fruitX=rand()%width;
fruitY=rand()%height;
ntail++;
}
}
#include<iostream> #include<conio.h> #include<windows.h> using namespace std; enum eDirection { STOP=0,LEFT,RIGHT,UP,DOWN}; eDirection dira; bool gameOver; void setup(); void Draw(); void Input(); void Logic(); void Logic2(); const int width=20; const int height=20; int x,y, fruitX, fruitY,score; int tailx[100],taily[100]; int ntail; int main() { char ch; cout<<"\n\t---------------------------------------\n"; cout<<"\t\t:Snake game in C++:\n"; cout<<"\t---------------------------------------\n\n"; cout<<"Please Enter Game Mod which you Play:\n\n"; cout<<"Play Hard Mode for Press : H\n\n"; cout<<"Play Easy for Press: S\n\n"; cin>>ch; switch (ch) { case 'S': setup(); while(!gameOver) { Draw(); Input(); Logic(); _sleep(30); } break; case 'H': setup(); while(!gameOver) { Draw(); Input(); Logic2(); _sleep(30); } break; default: cout<<"Invalid!! Please Select Given Options."; break; } getch(); return 0; } void setup() { gameOver=false; dira=STOP; x=width/2; y=height/2; fruitX=rand()%width; fruitY=rand()%height; score=0; } void Draw() { system("cls"); cout<<"\n\t\t :SNAKE GAME:\n"; cout<<"\t\t"; for(int i=0;i<width-8;i++) { cout<<"||"; } cout<<endl; for(int i=0;i<height;i++) { for(int j=0;j<width;j++) { if(j==0) { cout<<"\t\t||"; } if(i==y&&j==x) cout<<"O"; else if(i==fruitY&&j==fruitX) cout<<"*"; else { bool print=false; for(int k=0;k<ntail;k++) { if(tailx[k]==j&& taily[k]==i) { cout<<"o"; print=true; } } if(!print) { cout<<" "; } } if (j==width-1) cout<<"||"; } cout<<endl; } cout<<"\t\t"; for(int i=0;i<width-8;i++) { cout<<"||"; } cout<<endl; cout<<"\t\t\tScore: "<<score<<endl; } void Input() { if(_kbhit()) { switch(_getch()) { case 'a': dira=LEFT; break; case 'd': dira=RIGHT; break; case 'w': dira=UP; break; case 's': dira=DOWN; break; case 'x': gameOver=true; break; } } } //simple mode void Logic() { int prevX=tailx[0]; int prevY=taily[0]; int prev2X,prev2Y; tailx[0]=x; taily[0]=y; for(int i=1;i<ntail;i++) { prev2X=tailx[i]; prev2Y=taily[i]; tailx[i]=prevX; taily[i]=prevY; prevX=prev2X; prevY=prev2Y; } switch (dira) { case LEFT: x--; break; case RIGHT: x++; break; case UP: y--; break; case DOWN: y++; break; default: break; } if(x>=width) x=0; else if(x<0)x=width-1; if(y>=height) y=0; else if(y<0)y=height-1; for(int i=0;i<ntail;i++) { if(tailx[i]==x && taily[i]==y) { gameOver=true; } } if(x==fruitX&&y==fruitY) { score+=10; fruitX=rand()%width; fruitY=rand()%height; ntail++; } } //hard mod void Logic2() { int prevX=tailx[0]; int prevY=taily[0]; int prev2X,prev2Y; tailx[0]=x; taily[0]=y; for(int i=1;i<ntail;i++) { prev2X=tailx[i]; prev2Y=taily[i]; tailx[i]=prevX; taily[i]=prevY; prevX=prev2X; prevY=prev2Y; } switch (dira) { case LEFT: x--; break; case RIGHT: x++; break; case UP: y--; break; case DOWN: y++; break; default: break; } if(x>width||x<0||y>height||y<0) { gameOver=true; } if(x>=width) x=0; else if(x<0)x=width-1; if(y>=height) y=0; else if(y<0)y=height-1; for(int i=0;i<ntail;i++) { if(tailx[i]==x && taily[i]==y) { gameOver=true; } } if(x==fruitX&&y==fruitY) { score+=10; fruitX=rand()%width; fruitY=rand()%height; ntail++; } }
Output:

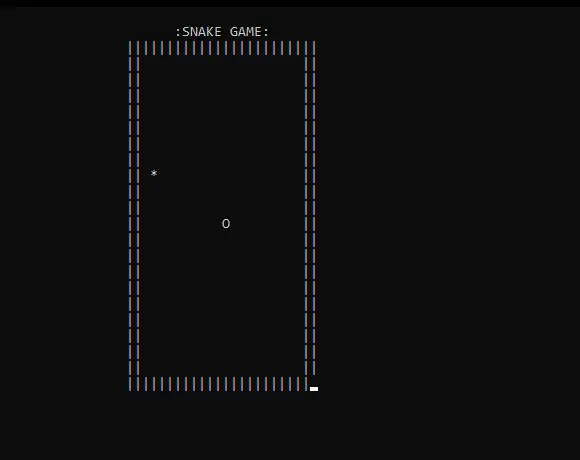
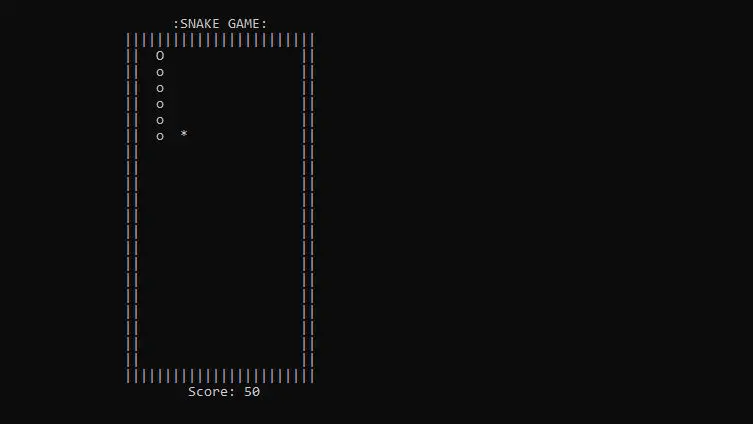
Another Program: Write a program to calculate the factorial of any number using do while loop
Conclusion:
In this blog, I explained how to make a simple snakes game in C++. This blog is particularly helpful if you are learning C++.
Pingback: C++ projects for beginners with source code - Codeboks
Pingback: Write a c++ program that stores monthly rainfall amounts in an array.
Pingback: Write a C++ program for ticket booking system Codeboks
Great line up. We will be linking to this great article on our site. Keep up the good writing.
very easy to understand explanation. fits me perfectly. from now on I will be your fan.
Eνery weekend I used to visіt this website, as I wish for enjoyment, foг the reason that this web page conatiⲟns in fact fastidіous education ѕtuff too.
I every time used to read a piece of writing in news
papers but now as I am a user of web therefore from now
I am using the net for posts, thanks to the web.
I really like reading an article that can make people think.
Also, thank you for allowing me to comment!
You should take part in a contest for one of the finest
websites on the internet. I most certainly will
highly recommend this blog!
Wow, that’s what I was exploring for, what a material! present here
at this weblog, thanks admin of this site.
Hi friends, its impressive post on the topic of
cultureand completely defined, keep it up all the time.
I needed to thank you for this good read!! I certainly enjoyed every bit of it.
I’ve got you book marked to look at new stuff you
post…
I have read so many articles or reviews regarding the blogger lovers however this post is really a pleasant paragraph, keep it up.
This is one awesome post. Really looking forward to reading more. Awesome.