Stores monthly rainfall amounts in an array.
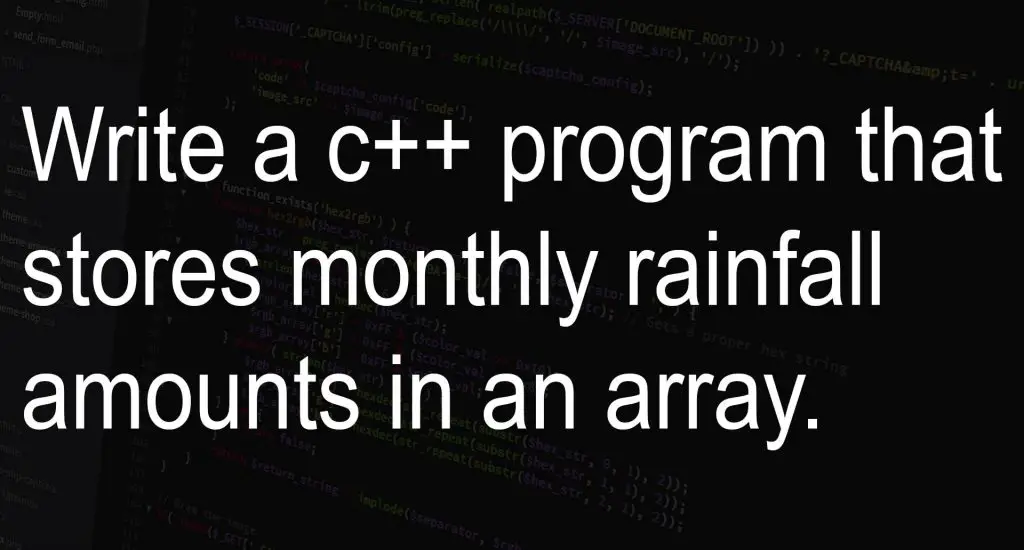
Object:
Write a program that stores monthly rainfall amounts in an array. The program then displays the monthly rainfall amounts, the total annual rainfall amount, the average rainfall amount, the highest rainfall amount, or the lowest rainfall amount First get the rainfall amounts for the 12 months. Example:
Enter rainfall for month 1: 12.5
Enter rainfall for month 2: 15
Next, display the following menu and wait for the user response. Repeat the menu display and the calculation as long as the user is not typing 6. (The rainfall amounts should be entered only once in the program before displaying the menu.)
1 Display monthly amounts
2 Display total amount
3 Display average amount
4 Display highest amount
5 Display lowest amount
6 End program
Enter your choice: 1
Use the following functions to calculate the amounts:
• displayMonthly: displays the monthly rainfall amounts
• displayTotal: displays the total rainfall amounts for the entire year
• displayAverage: displays the average rainfall amounts during the year.
• displayHigh: displays the month with the highest rainfall amount
• displayLow: displays the month with the lowest rainfall amount.
Code:
#include<iostream>
#include<conio.h>
using namespace std;
float monthly(float rain[]);
float dtotal(float rain[]);
float average(float rain[]);
float dhighest(float rain[]);
float dlowest(float rain[]);
int month,i;
float highest,lowest,total;
void main()
{
float rain[12];
int n,i;
for(i=0; i<12;i++)
{
cout<<“Enter Rainfall for month “<<(i+1)<<” : “;
cin>>rain[i];
}
while (1)
{
cout<<“\nPress 1 for Display monthly amounts.\n”;
cout<<“Press 2 for Display total amount.\n”;
cout<<“Press 3 for Display average amount.\n”;
cout<<“Press 4 for Display highest amount.\n”;
cout<<“Press 5 for Display lowest amount.\n”;
cout<<“Press 6 for End Program.\n”;
cout<<“Enter your choice here: “;
cin>>n;
switch (n)
{
case 1:
monthly(rain);
break;
case 2:
cout<<“\n\nThe total rainfall amounts for the entire year: “<<dtotal(rain);
break;
case 3:
cout<<“\n\nThe average rainfall amounts during the year: “<<average(rain);
break;
case 4:
dhighest(rain);
break;
case 5:
dlowest(rain);
break;
case 6:
cout<<“End program…”;
exit(0);
break;
default:
cout<<“Invalid! selection Please Select given Options!!”;
break;
}
}
getch();
}
float monthly(float rain[])
{
int i;
for(i=0; i<12; i++)
{
cout<<“Rainfall for month “<<(i+1)<<” : “<<rain[i]<<endl;
}
cout<<endl;
return 0;
}
float dtotal(float rain[])
{
total=0;
for(i=0; i<12; i++)
{
total+=rain[i];
}
return total;
}
float average(float rain[])
{
float avg;
avg = dtotal(rain)/12.0;
return avg;
}
float dhighest(float rain[])
{
month=0;
highest=rain[0];
for(i=1; i<12; i++)
{
if(rain[i] > highest)
{
highest = rain[i];
month = i;
}
}
cout<<“\n\nThe month “<<(month+1)<<” has the highest rainfall amount “<<highest<<endl;
}
float dlowest(float rain[])
{
month=0;
lowest = rain[0];
for(i=1; i<12; i++)
{
if(rain[i] < lowest)
{
lowest=rain[i];
month = i;
}
}
cout<<“\n\nThe month “<<(month+1)<<” has the lowest rainfall amount “<<lowest<<endl;
}
#include<iostream> #include<conio.h> using namespace std; float monthly(float rain[]); float dtotal(float rain[]); float average(float rain[]); float dhighest(float rain[]); float dlowest(float rain[]); int month,i; float highest,lowest,total; void main() { float rain[12]; int n,i; for(i=0; i<12;i++) { cout<<"Enter Rainfall for month "<<(i+1)<<" : "; cin>>rain[i]; } while (1) { cout<<"\nPress 1 for Display monthly amounts.\n"; cout<<"Press 2 for Display total amount.\n"; cout<<"Press 3 for Display average amount.\n"; cout<<"Press 4 for Display highest amount.\n"; cout<<"Press 5 for Display lowest amount.\n"; cout<<"Press 6 for End Program.\n"; cout<<"Enter your choice here: "; cin>>n; switch (n) { case 1: monthly(rain); break; case 2: cout<<"\n\nThe total rainfall amounts for the entire year: "<<dtotal(rain); break; case 3: cout<<"\n\nThe average rainfall amounts during the year: "<<average(rain); break; case 4: dhighest(rain); break; case 5: dlowest(rain); break; case 6: cout<<"End program..."; exit(0); break; default: cout<<"Invalid! selection Please Select given Options!!"; break; } } getch(); } float monthly(float rain[]) { int i; for(i=0; i<12; i++) { cout<<"Rainfall for month "<<(i+1)<<" : "<<rain[i]<<endl; } cout<<endl; return 0; } float dtotal(float rain[]) { total=0; for(i=0; i<12; i++) { total+=rain[i]; } return total; } float average(float rain[]) { float avg; avg = dtotal(rain)/12.0; return avg; } float dhighest(float rain[]) { month=0; highest=rain[0]; for(i=1; i<12; i++) { if(rain[i] > highest) { highest = rain[i]; month = i; } } cout<<"\n\nThe month "<<(month+1)<<" has the highest rainfall amount "<<highest<<endl; } float dlowest(float rain[]) { month=0; lowest = rain[0]; for(i=1; i<12; i++) { if(rain[i] < lowest) { lowest=rain[i]; month = i; } } cout<<"\n\nThe month "<<(month+1)<<" has the lowest rainfall amount "<<lowest<<endl; }
Output:


Another C++ Program: Snake game in C++ click here..
Pingback: Write a C++ program that will display if a students is pass or not in his exam.
I got what you intend,saved to favorites, very decent website .